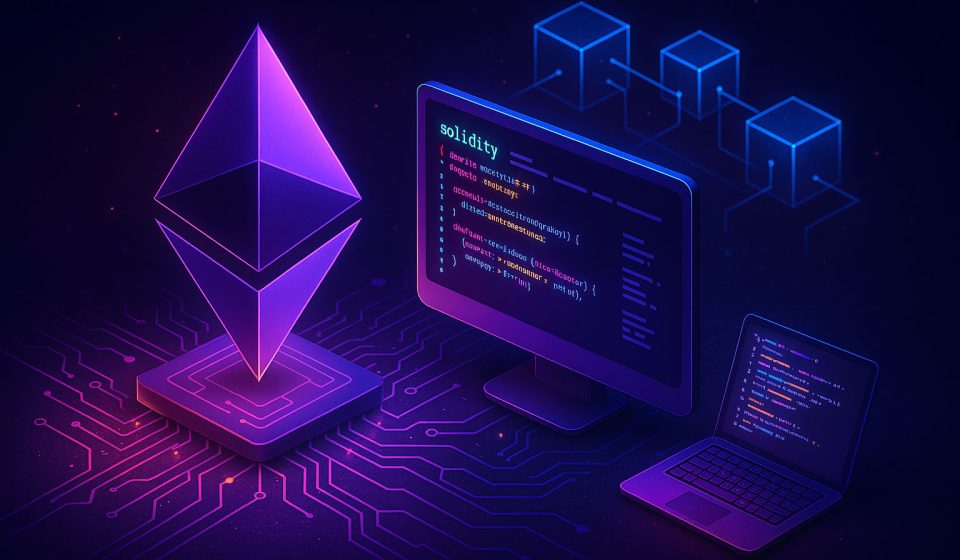
🧠 What Is Solidity? Getting Started with Ethereum Programming
Solidity is the go-to programming language for writing smart contracts on the Ethereum blockchain. If you’re curious about how to develop decentralized applications (DApps), automate blockchain transactions, or create your own token, then learning Solidity is your gateway into Ethereum programming.
Table Of Content
- 🧾 What Is Solidity?
- 🔑 Why Solidity Matters in Ethereum Programming
- 🛠️ Basic Solidity Syntax: A Quick Glimpse
- 🚀 How to Start Learning Solidity
- 🧰 Step 1: Use an Online IDE like Remix
- 📚 Step 2: Study the Solidity Docs
- 👨💻 Step 3: Practice with Tutorials & Courses
- ⚠️ Common Beginner Mistakes to Avoid
- 📈 What You Can Build with Solidity
- 🚪 Ready to Dive In?
- 🔍 Quick Recap
In this guide, we’ll break down what is Solidity, how it works, and how you can begin your journey into Ethereum development—even if you’re starting from scratch.
🧾 What Is Solidity?
Solidity is a high-level, object-oriented programming language designed specifically for writing smart contracts on Ethereum and other EVM-compatible blockchains. It was inspired by JavaScript, Python, and C++, making it relatively easy to pick up if you have prior coding experience.
Developed by the Ethereum Foundation in 2014, Solidity allows you to create self-executing contracts that run on the Ethereum Virtual Machine (EVM). Once deployed, these contracts cannot be altered—ensuring trustless, decentralized execution.
🔑 Why Solidity Matters in Ethereum Programming
Solidity is essential for Ethereum development because it enables:
- 🧱 Smart Contract Creation – Automate transactions, enforce rules, and build trustless systems.
- 🌐 DApp Development – Power decentralized applications in finance, gaming, identity, and more.
- 🪙 Token Standards – Deploy ERC-20, ERC-721 (NFTs), and ERC-1155 tokens.
- 🔐 Secure Logic – Create tamper-proof, permissionless digital contracts.
In short, Solidity is the foundation of most Ethereum-based innovations.
🛠️ Basic Solidity Syntax: A Quick Glimpse
Here’s a simple Solidity contract to show how it looks:
solidityCopyEdit// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract HelloWorld {
string public message = "Hello, Ethereum!";
function updateMessage(string memory _newMessage) public {
message = _newMessage;
}
}
🧪 This contract:
- Stores a public string message.
- Lets users update the message via a function.
It’s minimal but gives a taste of how smart contracts work.
🚀 How to Start Learning Solidity
Getting started with Solidity doesn’t require much—just the right tools and curiosity.
🧰 Step 1: Use an Online IDE like Remix
Try Remix, a browser-based environment where you can:
- Write Solidity code
- Compile contracts
- Deploy and test them on local or test networks
📚 Step 2: Study the Solidity Docs
The official Solidity documentation is a great place to dive deep into syntax, features, and best practices.
👨💻 Step 3: Practice with Tutorials & Courses
Explore beginner-friendly Solidity tutorials on:
⚠️ Common Beginner Mistakes to Avoid
When writing Solidity for the first time, watch out for:
- 🐛 Unprotected Functions – Always control access with
require()
andonlyOwner
modifiers. - 💸 Gas Inefficiencies – Avoid loops and optimize storage.
- 🔐 Security Flaws – Be aware of reentrancy attacks and check out OpenZeppelin for secure contract templates.
📈 What You Can Build with Solidity
Once you’ve mastered the basics, the possibilities are endless:
- 🪙 Launch your own cryptocurrency
- 🎮 Build blockchain games with NFTs
- 🏦 Create DeFi platforms for lending and staking
- 📃 Digitize legal contracts and enforce them automatically
Solidity puts you in control of the Ethereum ecosystem—and beyond.
🚪 Ready to Dive In?
Solidity is your entry point to the world of decentralized apps, financial protocols, NFT platforms, and more. Whether you’re an experienced developer or just getting started, Solidity opens the door to one of the most exciting revolutions in tech: blockchain development.
🔍 Quick Recap
- 🧠 Solidity is the main language for Ethereum smart contracts.
- 🔐 It allows decentralized, trustless, and secure applications.
- 🧰 Start with Remix, Solidity Docs, and simple contract exercises.
- ⚙️ Use best practices to build secure and efficient DApps.